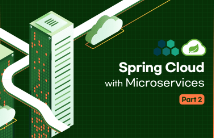
예를 들어 회원의 정보를 반환할 때,
해당 회원에 대한 상품 목록이 필요하다면
회원 서비스는 주문 서비스에 요청을 해야한다
Http Client
정보를 가져오는 것(GET)은 Http Client를 이용하며 크게 3가지 있다
- RestTemplate
- FeignClient
- WebClient
이 세가지는 다른 곳에도 많은 설명이 있기에 간단히만 짚고 넘어가자
RestTemplate
전통적인 Http Client, Deprecated 한다는 얘기가 있으나, 취소된 것으로 보인다 (토비님 영상[ https://youtu.be/S4W3cJOuLrU ])
FeignClient
넷플릭스에서 만든것이고 인터페이스로 보다 더 쉽게 사용할 수 있다
WebClient
앞선 두개와 달리 비동기 요청이 가능하다. WebFlux 스택이다
@LoadBalanced
org.springframework.cloud.client.loadbalancer의 @interface LoadBalanced를 사용하면 "localhost:8000" 등의 이름이 아닌 "/order-service" 로 마이크로 서비스 이름을 대신하여 사용할 수 있다
아래처럼 빈 등록시점에 어노테이션을 달면 된다
@LoadBalanced
@Bean
fun restTemplate() = RestTemplate()
@LoadBalance 주의사항
위 어노테이션을 사용하면 반드시 마이크로 서비스명을 사용해야한다, 그렇지 않으면 요청을 올바르게 보내지 않는다
(java.lang.IllegalArgumentException: Service Instance cannot be null)
예를 들면
"http://localhost:8000/order-service/~" 가 아니라
"http://order-service/order-service/~" 로 보내야 한다
저것 때문에 1시간 반이 날라갔다 ...
OpenFeign 로깅
@Bean
fun feignLoggerLevel(): Logger.Level = Logger.Level.FULL
logging.level:
com.example.userservice.httpclient: DEBUG
위 설정을 해주면 아래처럼 Http Client 호출을 볼 수 있다
이왕이면 예외처리도 해주도록 하자
private fun requestOrdersFromOrderServiceByFeignClient(userId: String): List<OrderResponse>? =
try {
orderServiceClient.requestOrders(userId)
} catch (ex: FeignException) {
log.error { ex.message }
null
}
OpenFeign 예외처리
OpenFeign의 ErrorDecoder를 이용하면, 예외처리를 할 수 있다,
URI가 잘못된 경우에 대해 상태 코드 및 메세지를 커스텀해보자
class FeignErrorDecoder : ErrorDecoder {
override fun decode(methodKey: String, response: Response): Exception? {
val exception = when (val statusCode: Int = response.status()) {
400 -> null
404 -> if (methodKey.contains("requestWrongOrders") || methodKey.contains("requestOrders")) {
ResponseStatusException(HttpStatus.valueOf(statusCode), "User's orders is empty!")
} else null
else -> Exception(response.reason())
}
return exception
}
}
그리고 저걸 Bean 등록해주면 된다
@Bean
fun feignErrorDecoder() = FeignErrorDecoder()
예외가 발생하면 디버깅에서 아래의 모습 확인할 수 있다
동기화 문제
(가정) User Service 하나와 Order Service 2개가 있다고 가정하자,
Order Service가 2개인 이유는 WAS 부하분산 목적이며 DB도 각각 하나씩 있다고 가정
주문건이 만일 한 사용자에 대해 Order Service #1이 1건, #2가 2건이라면... 곤란하게 될 것이다
동기화 문제를 막는 방법
- DB 저장소를 하나로 사용 (각 MS 마다)
- MQ Server 로 동기화
- Kafka Connector + DB 로 데이터 변경(CUD) 때마다 Queue로 DB 동기화
'Book & Lecture > 이도원님 MSA' 카테고리의 다른 글
Section 8: Cloud Bus (0) | 2023.05.28 |
---|---|
Section 7: Cloud Configuration (0) | 2023.05.24 |
Section 6: User Service (Security in Spring Boot 3.x) (삽질..) (0) | 2023.05.22 |
Section 3 ~ 5 E-Commerse App, User, Catalog, Orders MicroSerivce (0) | 2023.05.22 |
Secion 0~2: Micro Service와 Spring Cloud 소개, Service Discovery, API Gateway (0) | 2023.05.22 |
hi hello... World >< 가장 아름다운 하나의 해답이 존재한다
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!