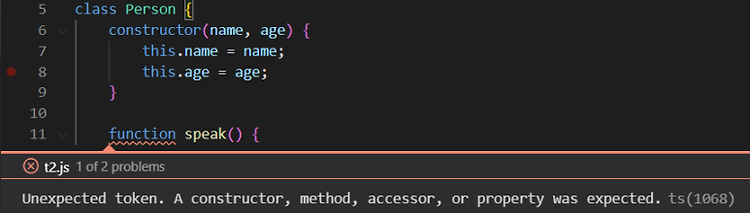
ellie : 함수.. 문법 정리Front-end/Vanilla JS2020. 11. 11. 21:30
Table of Contents
// Class ?
class Person {
constructor(name, age) {
this.name = name;
this.age = age;
}
speak() {
console.log(`${this.name}: hello!`);
}
}
const swCho = new Person('swCho', 20);
console.log(swCho);
클래스 내부에서 함수 정의시 function 예약어를 선언하지 않는군.. (method 등)
// Getter Setter : 캡슐화
debugger;
class User {
constructor(firstName, lastName, age) {
this.firstName = firstName;
this.lastName = lastName;
this.age = age;
}
get age() {
return this.age;
}
set age(value) {
this.age = value;
}
}
const swCho = new User('Seongwoo', 'Cho');
getter를 정의한 순간 this.age는 메모리의 데이터 읽어 오는 것이 아니라 getter를 호출
setter를 정의한 순간 = age, 값을 할당하는 과정에서 setter를 호출
무한루프를 타게 된다.. 잘 이해가 안돼니.. 나중에 다시 알아보도록 하고 밑에 기술하자..
숙제야.
getter setter 알아바 !
get age() {
return this._age;
}
set age(value) {
this._age = value;
}
...
set age(value) {
/*
if (value < 0) {
throw Error('age can not be negative');
}
*/
this._age = value < 0 ? 0 : value;
}
}
const swCho = new User('Seongwoo', 'Cho', -1);
console.log(swCho.age); // 0
그 외에 ES버젼이 높아짐에 따라 추가된 것들
예외 처리 해 주기..
#private, statis(싱글톤 등) ...
// Inheritance
class Shape {
constructor(width, height, color) {
this.width = width;
this.height = height;
this.color = color;
}
draw() {
console.log(`drawing ${this.color} color of`);
}
getArea() {
return this.width * this.height;
}
}
class Rectangle extends Shape {}
class Triangle extends Shape {
draw() {
console.log('🔺');
}
getArea() {
return (this.width * this.height) / 2;
}
}
const rectangle = new Rectangle(5, 8, 'blue');
rectangle.draw();
console.log(rectangle.getArea());
const triangle = new Triangle(5, 8, 'red');
triangle.draw();
console.log(triangle.getArea());
상속이야
// Class checking : instanceOf
console.log(rectangle instanceof Rectangle);
console.log(triangle instanceof Rectangle);
console.log(triangle instanceof Triangle);
console.log(triangle instanceof Shape);
console.log(triangle instanceof Object);
정답은 두번째것만 false
JavaScript reference
This part of the JavaScript section on MDN serves as a repository of facts about the JavaScript language. Read more about this reference.
developer.mozilla.org
참고하면 좋을 사이트
'Front-end > Vanilla JS' 카테고리의 다른 글
ellie: JSON (0) | 2020.11.11 |
---|---|
ellie : 객체 정리 (0) | 2020.11.11 |
Js 문법 객체 (0) | 2020.11.11 |
js 기초., 알게 된 것들... (0) | 2020.11.11 |
Js 문법 함수. ft. 클로저 (0) | 2020.10.15 |
@philo0407 :: Philo의 다락방
hi hello... World >< 가장 아름다운 하나의 해답이 존재한다
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!