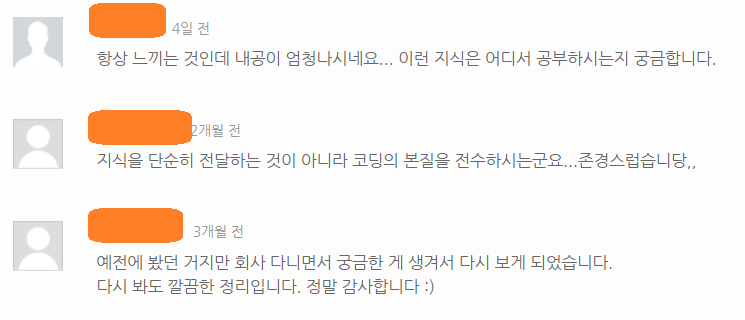
enum 그것이 알구싶다 !! 이넘아Back-end/Java, Kotlin2020. 12. 12. 21:43
Table of Contents
상수와 enum - 생활코딩 (opentutorials.org)
상수와 enum - 생활코딩
상수 상수는 변하지 않는 값이다. 아래에서 좌항이 변수이고 우항이 상수이다. int x = 1; 아래와 같은 구문은 있을 수 없다. 1은 2가 될 수 없다. 1 = 2; 상수의 이런 특성을 이용해서 아래와 같은 로
opentutorials.org
enum Fruit {
APPLE, BANANA, PEACH;
}
enum Company {
GOOGLE, APPLE, ORACLE;
}
public class enum배우자 {
public static void main(String[] args) {
Fruit type = Fruit.APPLE;
switch (type) {
case APPLE:
System.out.println(57);
break;
case BANANA:
System.out.println(34);
break;
case PEACH:
System.out.println(93);
break;
}
}
}
하아하아
내가 10개월 전에는 이해 못한.. enum을 지금에서야 이해한다 !!
우선 생활코딩 형님과 함께 enum으로 떠나는 여정을 살펴보장
public class ConstantDemo {
public static void main(String[] args) {
int type = 1;
switch(type){
case 1:
System.out.println(57);
break;
case 2:
System.out.println(34);
break;
case 3:
System.out.println(93);
break;
}
}
}
위 같은 경우는 주석이 필요하다.
public class enum배우자 {
int APPLE = 1, BANANA = 2, PEACH = 3;
int GOOGLE = 1, APPLE = 2, ORACLE = 3;
public static void main(String[] args) {
int type = APPLE;
switch(type){
case APPLE:
System.out.println(57);
break;
case BANANA:
System.out.println(34);
break;
case PEACH:
System.out.println(93);
break;
}
}
}
위 같은 경우도.. 당연히 컴파일 에러가 뜬다..
public class enum배우자 {
private final static int FRUIT_APPLE = 1;
private static final int FRUIT_BANANA = 2;
private static final int FRUIT_PEACH = 3;
private final static int COMPANY_GOOGLE = 1;
private static final int COMPANY_APPLE = 2;
private static final int COMPANY_ORACLE = 3;
public static void main(String[] args) {
if(Fruit.APPLE == Company.APPLE) {
out.println("과일애플과 기업애플은 같다");
}
int type = Fruit_APPLE;
switch(type){
case Fruit_APPLE:
System.out.println(57);
break;
case Fruit_BANANA:
System.out.println(34);
break;
case Fruit_PEACH:
System.out.println(93);
break;
}
}
}
자바의 문법으로 수정해 보자.
interface FRUIT {
int APPLE = 1, BANANA = 2, PEACH = 3;
}
interface COMPANY {
int GOOGLE = 2, APPLE = 1, ORACLE = 3;
}
public class enum배우자 {
public static void main(String[] args) {
if(Fruit.APPLE == Company.APPLE) {
out.println("과일애플과 기업애플은 같다");
}
int type = Fruit.APPLE;
switch(type){
case Fruit.APPLE:
System.out.println(57);
break;
case Fruit.BANANA:
System.out.println(34);
break;
case Fruit.PEACH:
System.out.println(93);
break;
}
}
}
런타임에서 벌어질 수 있는 의미상이 줄 수 있는 혼동을 컴파일 타임에 들고 와서
당초 컴파일이 못되게 하자.
class Fruit {
public final static Fruit APPLE = new Fruit();
public final static Fruit BANANA = new Fruit();
public final static Fruit PEACH = new Fruit();
}
class Company {
public final static Company GOOGLE = new Company();
public final static Company APPLE = new Company();
public final static Company ORACLE = new Company();
}
public class enum배우자 {
public static void main(String[] args) {
if(Fruit.APPLE == Company.APPLE) {
out.println("과일애플과 기업애플은 같다");
}
Fruit type = Fruit.APPLE;
switch(type){
case Fruit.APPLE:
System.out.println(57);
break;
case Fruit.BANANA:
System.out.println(34);
break;
case Fruit.PEACH:
System.out.println(93);
break;
}
}
}
위의 것도 컴파일이 되지 않는다. 이유는 switch에 들어가는 Data Type이 정해져 있기 때문
package practice;
import static java.lang.System.out;
import jdk.jfr.internal.PrivateAccess;
/*class Fruit {
public final static Fruit APPLE = new Fruit();
public final static Fruit BANANA = new Fruit();
public final static Fruit PEACH = new Fruit();
}*/
enum Fruit {
APPLE, BANANA, PEACH;
Fruit() {
out.println("Contructor of "+ this);
}
}
enum Company {
GOOGLE, APPLE, ORACLE;
}
public class enum배우자 {
public static void main(String[] args) {
out.println(1);
Fruit type = Fruit.APPLE;
out.println(2);
Fruit type2 = Fruit.APPLE;
out.println(3);
switch (type) {
case APPLE:
System.out.println(57);
break;
case BANANA:
System.out.println(34);
break;
case PEACH:
System.out.println(93);
break;
}
}
}
==== 결과 ====
1
Contructor of APPLE
Contructor of BANANA
Contructor of PEACH
2
3
57
위의 경우는 enum이 class와 같다는 것을 보여주고 있다.
enum Fruit {
APPLE("red"), BANANA("yellow"), PEACH("pink");
String color;
Fruit(String color) {
out.println("Contructor of "+ this);
this.color = color;
}
}
enum Company {
GOOGLE, APPLE, ORACLE;
}
public class enum배우자 {
public static void main(String[] args) {
Fruit type = Fruit.PEACH;
switch (type) {
case APPLE:
out.println(57);
out.println("color : "+ type.color);
break;
case BANANA:
out.println(34);
out.println("color : "+ Fruit.BANANA.color);
break;
case PEACH:
out.println(93);
out.println("color : "+ Fruit.PEACH.color);
break;
}
}
}
enum의 상수 안에는 값도 들어갈 수 있다.
캡슐화를 이용해서 자그마한 리팩터링을 해보자
enum Fruit {
APPLE("red"), BANANA("yellow"), PEACH("pink");
private String color;
Fruit(String color) {
out.println("Contructor of "+ this);
this.color = color;
}
public String getColor() {
return this.color;
}
}
enum Company {
GOOGLE, APPLE, ORACLE;
}
public class enum배우자 {
public static void main(String[] args) {
Fruit type = Fruit.PEACH;
switch (type) {
case APPLE:
out.println(57);
out.println("color : "+ type.getColor());
break;
case BANANA:
out.println(34);
out.println("color : "+ Fruit.BANANA.getColor());
break;
case PEACH:
out.println(93);
out.println("color : "+ Fruit.PEACH.getColor());
break;
}
}
}
enum이 갖고 있는 장점 하나 더
enum Fruit {
APPLE("red"), BANANA("yellow"), PEACH("pink");
private String color;
Fruit(String color) {
out.println("Contructor of "+ this);
this.color = color;
}
public String getColor() {
return this.color;
}
}
enum Company {
GOOGLE, APPLE, ORACLE;
}
public class enum배우자 {
public static void main(String[] args) {
for (Fruit val : Fruit.values()) {
out.println(val);
}
}
}
class와 달리 순회할 수 있다는 장점이 있다.
'Back-end > Java, Kotlin' 카테고리의 다른 글
싱글톤 패턴에 객체 주입받기! (ft.빌더) (0) | 2021.03.07 |
---|---|
디자인패턴 중.. State (2) | 2020.12.17 |
다형성과 형변환, instance : 네이버 블로그 (0) | 2020.10.01 |
Java.. ArrayList안에 ArrayList만들기 : 네이버 블로그 (0) | 2020.10.01 |
작은 알아낸 것들 : 네이버 블로그 (0) | 2020.10.01 |
@philo0407 :: Philo의 다락방
hi hello... World >< 가장 아름다운 하나의 해답이 존재한다
포스팅이 좋았다면 "좋아요❤️" 또는 "구독👍🏻" 해주세요!